TIL 3応答-ライフサイクル
LifreCyle
Reactを使用する場合、構成部品をクラスまたは関数として定義できます.構成部品には、プロパティポイントでコードに火をつけるを実行するように設定できる複数のライフサイクルメソッドがあります.これを
Life Cycle Event
と呼びます.
各ステップで実行する方法を見てみましょう.
Mounting
素子の初回動作時を
Mount
と呼ぶ.constructor()
私たちが作成した構成部品がブラウザに表示されたときに最初に実行される関数です.1回のみ実行され、コンストラクション関数の内部で宣言されたsetStateは無視されたフィーチャーを有します.
constructor(props) {
super(props);
console.log('constructor');
}
render()
シンボルをレンダリングします.
componentDidMount()
構成部品を作成しrenderを呼び出して実行する方法.
componentDidMount() {
console.log('componentDidMount');
}
Updating
static getDerivedStateFromProps
v16.3
からcomponentWillReceiveProps
APIを使用せずにgetDerivedStateFromsProps
が生成される.props値とstate値をフリーズするために使用します.//MyComponent.js
import React, { Component } from "react";
class MyComponent extends Component {
state = {
value: 0,
};
static getDerivedStateFromProps(nextProps, prevState) {
if (prevState.value !== nextProps.value) {
return {
value: nextProps.value,
};
}
return null;
}
render() {
return (
<div>
<p>props: {this.props.value}</p>
<p>state: {this.state.value}</p>
</div>
);
}
}
export default MyComponent;
//App.js
import React, { Component } from 'react';
import MyComponent from 'Mycomponent';
class App extends Component {
state = {
counter: 1
}
constructor(props) {
super(props);
}
handleClick = () => {
this.setState({
counter: this.state.counter + 1
});
}
render() {
return (
<div>
<MyComponent value={this.state.counter}/>
<button onClick={this.handleClick}>Click Me</button>
</div>
);
}
}
上記のコードを実行する場合、ボタンをクリックしてpropsの値を状態値の変化に伴って1増加させます.sholudComponentUpdate
sholudComponentUpdate
は、更新を防止することができる.特定の条件に従ってレンダリングをブロックします.//MyComponent.js
import React, { Component } from "react";
class MyComponent extends Component {
state = {
value: 0,
};
static getDerivedStateFromProps(nextProps, prevState) {
if (prevState.value !== nextProps.value) {
return {
value: nextProps.value,
};
}
return null;
}
//추가 된 부분
shouldComponentUpdate(nextProps, nextState) {
if (nextProps.value === 10) return false;
return true;
}
render() {
return (
<div>
<p>props: {this.props.value}</p>
<p>state: {this.state.value}</p>
</div>
);
}
}
export default MyComponent;
条件文のprops値が10の場合、画面には何も表示されません(画面には何も表示されません).getSnapshotBeforeUpdate
DOMが変化する前のDOM状態を取得する.この部分は例で分かりやすい.
componentDidUpdate
特定のpropsの値が変化すると、特定の操作を実行できます.API呼び出しは通常、このオブジェクトを必要とするので、
componentDidUpdate
の方法で処理することができる.以前のprops値と現在の値が変更された場合、コンソールにメッセージが表示されます.//MyComponent.js
import React, { Component } from "react";
class MyComponent extends Component {
state = {
value: 0,
};
static getDerivedStateFromProps(nextProps, prevState) {
if (prevState.value !== nextProps.value) {
return {
value: nextProps.value,
};
}
return null;
}
shouldComponentUpdate(nextProps, nextState) {
if (nextProps.value === 10) return false;
return true;
}
//추가된 부분
componentDidUpdate(preProps, prevState) {
if(this.props.value !== prevProps.value){
console.log('value 값이 바뀌었습니다.', this.props.value);
}
}
render() {
return (
<div>
<p>props: {this.props.value}</p>
<p>state: {this.state.value}</p>
</div>
);
}
}
export default MyComponent;
Unmounting
componentWillUnmount
この関数は、特定の条件で構成部品を削除します.値が10の場合、MyComponent素子が消えてしまう例.
//App.js
import React, { Component } from 'react';
import MyComponent from 'Mycomponent';
class App extends Component {
state = {
counter: 1
}
constructor(props) {
super(props);
}
handleClick = () => {
this.setState({
counter: this.state.counter + 1
});
}
render() {
return (
<div>
{this.state.counter < 10 && < MyComponent value={this.state.counter}/>}
<button onClick={this.handleClick}>Click Me</button>
</div>
);
}
}
//MyComponent.js
import React, { Component } from "react";
class MyComponent extends Component {
state = {
value: 0,
};
static getDerivedStateFromProps(nextProps, prevState) {
if (prevState.value !== nextProps.value) {
return {
value: nextProps.value,
};
}
return null;
}
shouldComponentUpdate(nextProps, nextState) {
if (nextProps.value === 10) return false;
return true;
}
componentDidUpdate(preProps, prevState) {
if(this.props.value !== prevProps.value){
console.log('value 값이 바뀌었습니다.', this.props.value);
}
}
//추가된 부분
componentWillUnmount() {
console.log('ByeBye');
}
render() {
return (
<div>
<p>props: {this.props.value}</p>
<p>state: {this.state.value}</p>
</div>
);
}
}
export default MyComponent;
componentDidCatch
render()エラーが発生した場合、この関数を処理できます.たとえば、ゲーム中にエラーで異常終了した場合、次のメッセージが表示されます.この場合、「レポート」をクリックして開発者にエラーに関する情報を送信します.開発者は、取得されていないエラーを誤って取得する可能性があります.重要な点は、エラーが発生する可能性のある構成部品の親構成部品で使用できることです.
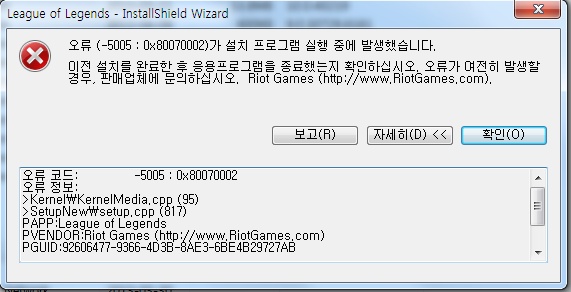
Reference
この問題について(TIL 3応答-ライフサイクル), 我々は、より多くの情報をここで見つけました https://velog.io/@wndud0647/TIL-3-React-생명주기LifeCycleテキストは自由に共有またはコピーできます。ただし、このドキュメントのURLは参考URLとして残しておいてください。
Collection and Share based on the CC Protocol