実際のJavaScriptアレイ法
34199 ワード
これは、JavaScriptの配列メソッドを使用する方法を示す例で、ステップバイステップのチュートリアルです.
フィルタアレイ法
マップ法
foreachメソッド

EX 2

EX 3 .

EX 4 .

EX 5 .

Ex . 6
配列メソッド

解説
マップアレイ法

アレイメソッド

すべての配列メソッド

解説
配列法

解説
アレイメソッドを減らす

解説
この情報が役に立つことを願っています.
他の配列メソッドは、どのようにそれらを使用するかの例とともに、コメントセクションに残しておくべきです.
フィルタアレイ法
const laptop = [
{
model: 'Macbook Pro',
price: 20000,
},
{
model: 'Macbook Air',
price: 15000,
},
{
model: 'Hp lite',
price: 5000,
},
{
model: 'Dell',
price: 400,
},
];
const filteredLaptop = laptop.filter((laptop) => {
return laptop.price > 10000;
});
console.log(filteredLaptop);
解説1. We are creating a new array called filteredLaptop.
2. We are filtering through the array laptop and checking if the laptop.price is greater than 10000.
3. If so, we are adding the laptop to the filteredLaptop array.
4. We then return filteredLaptop.
マップ法
const laptop = [
{
model: 'Macbook Pro',
price: 20000,
},
{
model: 'Macbook Air',
price: 15000,
},
{
model: 'Hp lite',
price: 5000,
},
{
model: 'Dell',
price: 400,
},
];
const getLaptopModel = laptop.map((item) => {
return item.model;
});
console.log(getLaptopModel);
解説1. We create an array with 3 objects inside.
2. We create an arrow function to map the array and return the property of model.
3. We log the result.
foreachメソッド
const countries = [
'Nigeria',
'Ghana',
'Haiti',
'Jamaica',
'Kenya',
'Tanzania',
'Uganda',
'Zambia',
'Zimbabwe',
];
//Ex 1
countries.forEach((country, index) => {
console.log(`I am from ${country} and my index is ${index}`);
// foreach does not return anything o
});
結果:EX 2
countries.forEach((country, index) => {
if (country === 'Zambia') {
console.log(`I am from ${country} and my index is ${index}`);
}
});
結果:EX 3 .
countries.forEach((country, index) => {
if (country.length > 7) {
console.log(`${country} character is greater than 7`);
}
});
結果:EX 4 .
countries.forEach((country, index) => {
let containedLetters = 'han';
if (country.includes(containedLetters)) {
console.log(`${country} contains the letter ${containedLetters}`);
}
});
結果:EX 5 .
countries.forEach((country, index) => {
let containedLetters = 'Nig';
if (country.startsWith(containedLetters)) {
console.log(`${country} Starts with the letter ${containedLetters}`);
}
});
結果:Ex . 6
const countries = [
{ name: 'Nigeria', landMass: '3000' },
{ name: 'Ghana', landMass: '787878' },
{ name: 'Haiti', landMass: '293829' },
{ name: 'Jamaica', landMass: '666660' },
{ name: 'Kenya', landMass: '8934' },
{ name: 'Tanzania', landMass: '3434.5' },
{ name: 'Uganda', landMass: '23232' },
{ name: 'Zambia', landMass: '4444' },
{ name: 'Zimbabwe', landMass: '111' },
];
countries.forEach((country, index) => {
console.log(country.name);
Result:
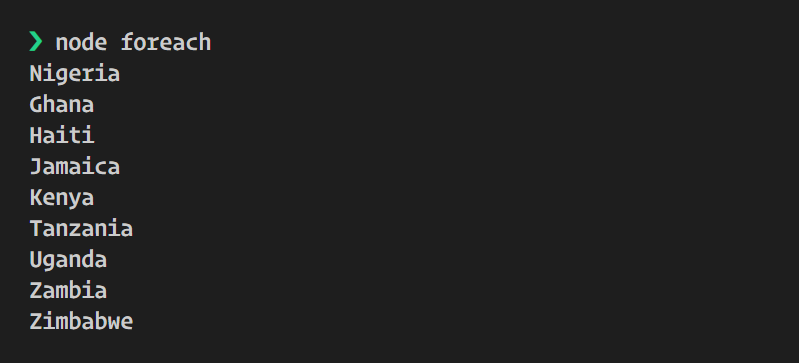
});
配列メソッド
const items = [1, 2, 3, 4, 5, 6];
const includesTwo = items.includes(2);
console.log(includesTwo);
結果:解説
1. In Javascript, the includes method is a method from the Array prototype
2. This method takes an argument and returns true or false depending on whether the argument is in the Array or not
マップアレイ法
const laptop = [
{
model: 'Macbook Pro',
price: 20000,
},
{
model: 'Macbook Air',
price: 15000,
},
{
model: 'Hp lite',
price: 5000,
},
{
model: 'Dell',
price: 400,
},
];
const getLaptopModel = laptop.map((item) => {
return item.model;
});
console.log(getLaptopModel);
結果:アレイメソッド
//Find array method
const laptop = [
{
model: 'Macbook Pro',
price: 20000,
},
{
model: 'Macbook Air',
price: 15000,
},
{
model: 'Hp lite',
price: 5000,
},
{
model: 'Dell',
price: 400,
},
];
const findLaptop = laptop.find((laptop) => {
return laptop.model === 'Macbook Pro';
});
console.log(findLaptop);
結果:すべての配列メソッド
const food = [
{
name: 'pizza',
price: 100,
},
{
name: 'burger',
price: 200,
},
{
name: 'pasta',
price: 300,
},
{
name: 'rice',
price: 400,
},
{
name: 'chicken',
price: 500,
},
];
const checkIfFoodHasPriceLessThan200 = food.every((item) => {
return item.price < 200;
});
console.log(checkIfFoodHasPriceLessThan200);
結果:解説
1. food is an array of objects.
2. food.every() is a method on the array.
3. Every() method iterates over each item in the array, and calls the function
passed to it. The function is passed the item, and the index of that item.
If the function returns true, the every() method returns true, otherwise
every() returns false.
4. The function checks if the price of each item is less than 200.
5. checkIfFoodHasPriceLessThan200 is set to true if every item has a price
less than 200, otherwise it returns false.
配列法
const food = [
{
name: 'pizza',
price: 100,
},
{
name: 'burger',
price: 200,
},
{
name: 'pasta',
price: 300,
},
{
name: 'rice',
price: 400,
},
{
name: 'chicken',
price: 500,
},
];
const checkIfFoodHasPriceLessThan200 = food.some((item) => {
return item.price < 200;
});
console.log(checkIfFoodHasPriceLessThan200);
結果:解説
1. The code checks if any item in the food array has a price less than 200.
2. The .some() method checks if any of the elements in the food array returns true.
3. The .some() method returns true if any of the elements in the food array return true.
4. The .some() method returns false if all of the elements in the food array return false.
アレイメソッドを減らす
const food = [
{
name: 'pizza',
price: 100,
},
{
name: 'burger',
price: 200,
},
{
name: 'pasta',
price: 300,
},
{
name: 'rice',
price: 400,
},
{
name: 'chicken',
price: 500,
},
];
const total = food.reduce((currentTotal, item) => {
return item.price + currentTotal;
}, 0);
console.log(total);
結果:解説
1. We are using the reduce function to add up the total price of all the food items in the array.
2. The first argument of the reduce function is the currentTotal.
3. The second argument of the reduce function is the item.
4. We are using the item.price to add the value of item.price to the currentTotal.
5. The currentTotal is set to 0 because we want to start with a base value of 0.
6. The value of currentTotal is returned at the end of the reduce function.
7. The value of the total is logged to the console.
結論
この情報が役に立つことを願っています.
他の配列メソッドは、どのようにそれらを使用するかの例とともに、コメントセクションに残しておくべきです.
Reference
この問題について(実際のJavaScriptアレイ法), 我々は、より多くの情報をここで見つけました https://dev.to/drsimplegraffiti/practical-javascript-array-methods-4nnkテキストは自由に共有またはコピーできます。ただし、このドキュメントのURLは参考URLとして残しておいてください。
Collection and Share based on the CC Protocol